Простое слайдшоу
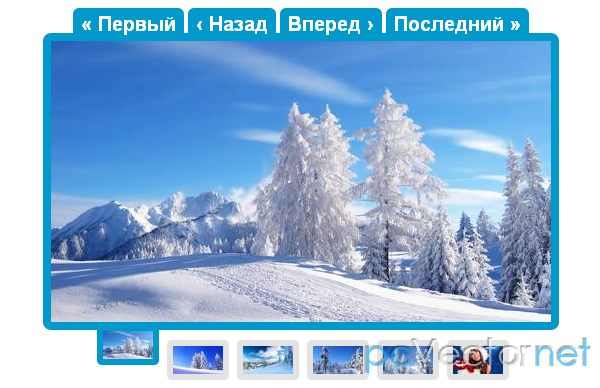
Простенькое слайдшоу на jquery.
HTML
В демке используется 6 полноразмерных изображений и 6 миниатюр:
<div id="container">
<div id="navigate">
<a href="" id="linkfirst">« Первый</a>
<span id="spanfirst">« Первый</span>
<a href="" id="linkprev">‹ Назад</a>
<span id="spanprev">‹ Назад</span>
<a href="" id="linknext">Вперед ›</a>
<span id="spannext">Вперед ›</span>
<a href="" id="linklast">Последний »</a>
<span id="spanlast">Последний »</span>
</div>
<div id="img_container">
<div id="img_box">
<img id="img1" src="img/original/01.jpg"/>
<img id="img2" src="img/original/02.jpg"/>
<img id="img3" src="img/original/03.jpg"/>
<img id="img4" src="img/original/04.jpg"/>
<img id="img5" src="img/original/05.jpg"/>
<img id="img6" src="img/original/06.jpg"/>
<div style="clear:both;"></div>
</div>
</div>
<div id="imgthumb_box">
<a href="" class="thumblink" id="imglink1"><img src="img/thumb/01.jpg"/></a>
<a href="" class="thumblink" id="imglink2"><img src="img/thumb/02.jpg"/></a>
<a href="" class="thumblink" id="imglink3"><img src="img/thumb/03.jpg"/></a>
<a href="" class="thumblink" id="imglink4"><img src="img/thumb/04.jpg"/></a>
<a href="" class="thumblink" id="imglink5"><img src="img/thumb/05.jpg"/></a>
<a href="" class="thumblink" id="imglink6"><img src="img/thumb/06.jpg"/></a>
</div>
</div>
CSS
Обратите внимание на то, чтобы #img_container содержал свойство overflow: hidden; - чтобы картинки были только внутри слайдера:
body {
margin: 0;
padding: 0;
font-family: Arial, "Free Sans";
}
#main {
background: #0099cc;
margin-top: 0;
padding: 2px 0 4px 0;
text-align: center;
}
#main a {
color: #ffffff;
text-decoration: none;
font-size: 12px;
font-weight: bold;
font-family: Arial;
}
#main a:hover {
text-decoration: underline;
}
#container {
margin-top: 40px;
}
#navigate {
text-align: center;
}
#navigate a, span {
position: relative;
top: 3px;
background: #0099cc;
text-decoration: none;
color: #fff;
padding: 4px 8px 0 8px;
font-size: 20px;
font-weight: bold;
-webkit-border-radius: .3em .3em 0 0;
-moz-border-radius: .3em .3em 0 0;
border-radius: .3em .3em 0 0;
}
#navigate a:hover {
color: #d3d3d3;
}
#navigate span {
display: none;
color: #999;
}
#img_container {
overflow: hidden;
width: 500px;
margin: 0 auto;
border: 8px solid #0099cc;
-webkit-border-radius: .5em;
-moz-border-radius: .5em;
border-radius: .5em;
}
#img_box {
position: relative;
width: 3000px;
margin: 0;
}
#img_box img {
float: left;
}
#imgthumb_box {
text-align: center;
}
#imgthumb_box a {
margin-left: 4px;
}
#imgthumb_box a img {
border: 6px solid #e3e3e3;
position: relative;
top: 10px;
-webkit-border-radius: .3em;
-moz-border-radius: .3em;
border-radius: .3em;
}
#imgthumb_box a img:hover {
border-color: #666;
}
JS
Подключаем jQuery и скрипт:
<script type="text/jаvascript" src="http://ajax.googleapis.com/ajax/libs/jquery/1.7.1/jquery.min.js"></script>
<script type="text/jаvascript">
$(document).ready(function() {
var total = $("#img_box img").length;
$("#imglink1 img").css({
"border-color": "#0099cc",
"top": "-5px"
});
$(".thumblink").click(function() {
var imgnumber = parseInt($(this).attr('id').replace("imglink", ""));
var move = -($("#img"+imgnumber).width() * (imgnumber - 1));
$("#img_box").animate({
left: move
}, 500);
$("#imgthumb_box").find("img").removeAttr("style");
$(this).find("img").css({
"border-color": "#0099cc",
"top": "-5px",
"border-top-width": "-5px"
});
return false;
});
$("#navigate a").click(function() {
var imgwidth = $("#img1").width();
var box_left = $("#img_box").css("left");
var el_id = $(this).attr("id");
var move, imgnumber;
if (box_left == 'auto') {
box_left = 0;
} else {
box_left = parseInt(box_left.replace("px", ""));
}
// if prev
if (el_id == 'linkprev') {
if ((box_left - imgwidth) == -(imgwidth)) {
move = -(imgwidth * (total - 1));
} else {
move = box_left + imgwidth;
}
imgnumber = -(box_left / imgwidth);
if (imgnumber == 0) {
imgnumber = total;
}
} else if (el_id == 'linknext') {
// if in the last image, move to first
if (-(box_left) == (imgwidth * (total - 1))) {
move = 0;
} else {
move = box_left - imgwidth;
}
imgnumber = Math.abs((box_left / imgwidth)) + 2;
if (imgnumber == (total + 1)) {
imgnumber = 1;
}
} else if (el_id == 'linkfirst') {
move = 0;
imgnumber = 1;
} else if (el_id == 'linklast') {
move = -(imgwidth * (total - 1));
imgnumber = total;
}
// styling selected image
$("#imgthumb_box").find("img").removeAttr("style");
$("#imglink"+imgnumber).find("img").css({
"border-color": "#0099cc",
"top": "-5px",
"border-top-width": "-5px"
});
$("#navigate a").hide();
$("#navigate span").show();
$("#img_box").animate({
left: move+'px'
}, 400, function() {
$("#navigate a").show();
$("#navigate span").hide();
});
return false;
});
});
</script>
Ссылки