Оригинальные часы
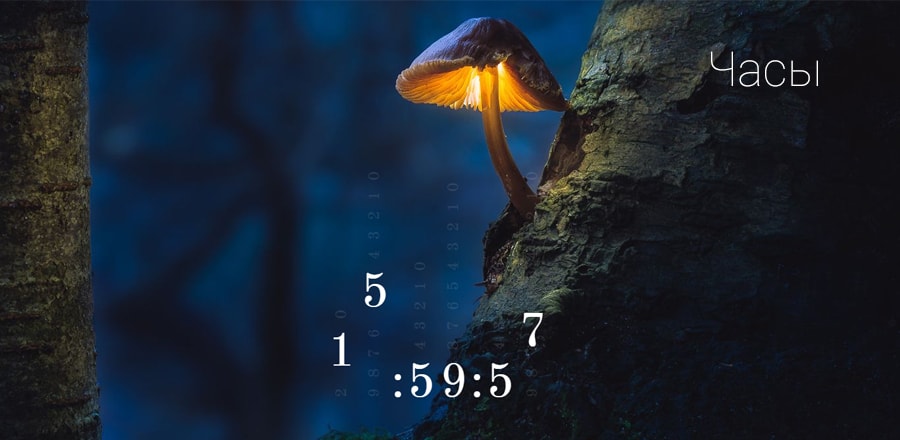
JS
Скрипт часов (написан на ES6)let _timeElement10Digits = Array.from(Array(10)).map((n, i) => i);
let _timeElement6Digits = _timeElement10Digits.slice(0, 6);
let _timeElement3Digits = _timeElement10Digits.slice(0, 3);
let _timeElementStructure = [
[ _timeElement3Digits, _timeElement10Digits ],
[ _timeElement6Digits, _timeElement10Digits ],
[ _timeElement6Digits, _timeElement10Digits ]
];
let clock = document.createElement('div');
clock.id = 'clock';
document.body.appendChild(clock);
let digitGroups = [];
requestAnimationFrame(update);
_timeElementStructure.forEach(digits => {
let digitGroup = document.createElement('div');
digitGroup.classList.add('digit-group');
clock.appendChild(digitGroup);
digitGroups.push(digitGroup);
digits.forEach(digitList => {
let digit = document.createElement('div');
digit.classList.add('digit');
digitList.forEach(n => {
let ele = document.createElement('div');
ele.classList.add('digit-number');
ele.innerText = n;
digit.appendChild(ele);
});
digitGroup.appendChild(digit);
});
});
function update() {
requestAnimationFrame(update);
let date = new Date();
let time = [ date.getHours(), date.getMinutes(), date.getSeconds() ]
.map(n => `0${n}`.slice(-2).split('').map(e => +e))
.reduce((p, n) => p.concat(n), []);
time.forEach((n, i) => {
let digit = digitGroups[Math.floor(i * 0.5)].children[i % 2].children;
Array.from(digit).forEach((e, i2) => e.classList[i2 === n ? 'add' : 'remove']('highlight'));
});
}
CSS
Для цифр используется один из google шрифтов@import url("https://fonts.googleapis.com/css?family=Old+Standard+TT");
html,
body {
height: 100%;
font-family: 'Old Standard TT', serif;
background-color: #1f1f1f;
margin: 0;
font-size: 0;
color: white;
text-align: center;
overflow: hidden;
background-image: url(../img/bg.jpg);
-webkit-background-size: cover;
background-size: cover;
background-repeat: no-repeat;
background-position: center bottom;
}
#clock {
font-size: 24px;
width: 350px;
height: 350px;
position: absolute;
left: 50%;
top: 50%;
margin-left: -175px;
margin-top: -175px;
}
.digit-group {
display: inline-block;
height: 350px;
}
.digit-group:not(:last-child):after {
content: ':';
font-size: 72px;
}
.digit {
display: inline-block;
width: 50px;
height: 350px;
}
.digit .digit-number {
color: rgba(255, 255, 255, 0.1);
-webkit-transform: rotate(-90deg);
transform: rotate(-90deg);
-webkit-transition: font-size 200ms, color 150ms, -webkit-transform 350ms;
transition: font-size 200ms, color 150ms, -webkit-transform 350ms;
transition: font-size 200ms, transform 350ms, color 150ms;
transition: font-size 200ms, transform 350ms, color 150ms, -webkit-transform 350ms;
}
.digit .digit-number.highlight {
color: inherit;
font-size: 72px;
-webkit-transform: rotate(0deg);
transform: rotate(0deg);
}