Фрактальные круги
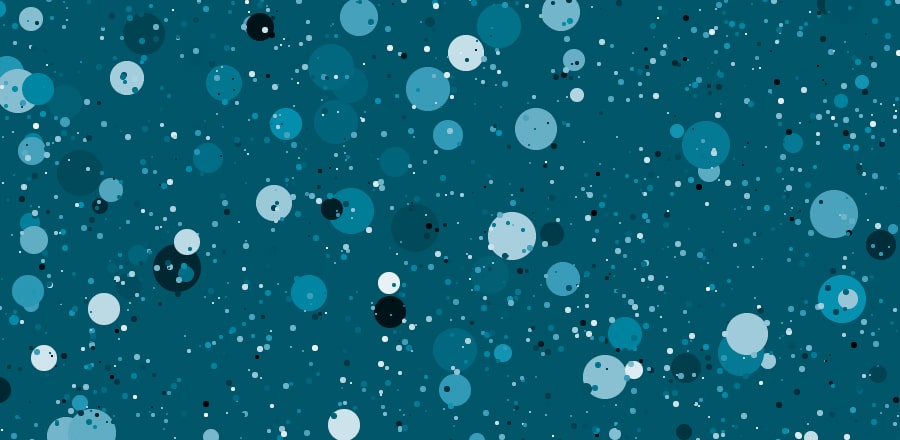
HTML
<canvas id="canvas"></canvas>
CSS
body{
background: #232323;
overflow: hidden;
padding: 0px;
margin: 0px;
}
#canvas{
width: 100%;
height: 100%;
}
#info{
font-size: 20px;
position: absolute;
top: 5px;
left: 5px;
background: #000;
color: #fff;
padding: 5px;
border-radius: 5px;
}
JS
Подключаем jQuery:https://cdnjs.cloudflare.com/ajax/libs/jquery/3.2.1/jquery.min.js
скриптvar canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
ctx.canvas.width = window.innerWidth;
ctx.canvas.height = window.innerHeight;
pCW = ctx.canvas.width / 100;
pCWmin = Math.floor(pCW * 45);
pCWmax = Math.floor(pCW * 55);
pCH = ctx.canvas.height / 100;
pCHmin = Math.floor(pCH * 45);
pCHmax = Math.floor(pCH * 55);
$(window).on("resize", function() {
ctx.canvas.width = window.innerWidth;
ctx.canvas.height = window.innerHeight;
});
// Circle Sizes
maxRadiusNano = 3;
minRadiusNano = 1;
maxRadiusSmall = 20;
minRadiusSmall = 5;
maxRadiusBig = 150;
minRadiusBig = 100;
// Amount of Circles
maxCirclesNano = 5000;
maxCirclesSmall = 300;
maxCirclesBig = 5;
// Offset for Lightness (HSL)
colOffset = 50;
H = Math.floor(Math.random() * 361) + 0;
S = Math.floor(Math.random() * 60) + 20;
L = Math.floor(Math.random() * 60) + 20;
$("#canvas").css({background: `hsl(${H}, ${S}%, ${L}%)`});
function drawCircleBig() {
radius = Math.floor((Math.random() * maxRadiusBig) + minRadiusBig);
xPos = Math.floor((Math.random() * pCWmax) + pCWmin) - radius/2;
yPos = Math.floor((Math.random() * pCHmax) + pCHmin) - radius/2;
var Lo = Math.floor((Math.random() * colOffset) + 1);
if(L < 50){L = L + Lo} else {L = L - Lo};
var HSL = `hsl(${H}, ${S}%, ${L}%)`;
ctx.beginPath();
ctx.fillStyle = HSL;
ctx.arc(xPos, yPos, radius, 0, 2 * Math.PI);
ctx.fill();
ctx.closePath();
};
function drawCircleSmall() {
radius = Math.floor((Math.random() * maxRadiusSmall) + minRadiusSmall);
xPos = Math.floor((Math.random() * window.innerWidth) + radius);
yPos = Math.floor((Math.random() * window.innerHeight) + radius);
var Lo = Math.floor((Math.random() * colOffset) + 1);
if(L < 50){L = L + Lo} else {L = L - Lo};
var HSL = `hsl(${H}, ${S}%, ${L}%)`;
ctx.beginPath();
ctx.fillStyle = HSL;
ctx.arc(xPos, yPos, radius, 0, 2 * Math.PI);
ctx.fill();
ctx.closePath();
};
function drawCircleNano() {
radius = Math.floor((Math.random() * maxRadiusNano) + minRadiusNano);
xPos = Math.floor((Math.random() * window.innerWidth) + radius);
yPos = Math.floor((Math.random() * window.innerHeight) + radius);
var Lo = Math.floor((Math.random() * colOffset) + 1);
if(L < 50){L = L + Lo} else {L = L - Lo};
var HSL = `hsl(${H}, ${S}%, ${L}%)`;
ctx.beginPath();
ctx.fillStyle = HSL;
ctx.arc(xPos, yPos, radius, 0, 2 * Math.PI);
ctx.fill();
ctx.closePath();
};
$("#canvas").on("click", function(){
H = Math.floor((Math.random() * 361) + 0);
S = Math.floor((Math.random() * 40) + 30);
L = Math.floor((Math.random() * 40) + 30);
$("#canvas").css({background: `hsl(${H}, ${S}%, ${L}%)`});
ctx.canvas.width = window.innerWidth;
ctx.canvas.height = window.innerHeight;
ctx.clearRect(0,0,ctx.canvas.width,ctx.canvas.height)
for(i = 0; i < maxCirclesBig; i++){drawCircleBig();}
for(i = 0; i < maxCirclesSmall; i++){drawCircleSmall();}
for(i = 0; i < maxCirclesNano; i++){drawCircleNano();}
});
for(i = 0; i < maxCirclesBig; i++){drawCircleBig();}
for(i = 0; i < maxCirclesSmall; i++){drawCircleSmall();}
for(i = 0; i < maxCirclesNano; i++){drawCircleNano();}